Welcome to the world of Python programming!
If you are a beginner looking to level up your skills and take the next step in your Python journey, you have come to the right place. Python is a versatile language used in a wide range of applications such as web development, machine learning, data analysis, and more. With its simple syntax and easy-to-learn structure, Python is an excellent language for beginners to start their programming journey.
If you are a beginner looking to level up your skills and take the next step in your Python journey, you have come to the right place. Python is a versatile language used in a wide range of applications such as web development, machine learning, data analysis, and more. With its simple syntax and easy-to-learn structure, Python is an excellent language for beginners to start their programming journey.
In this article, we’ll take a look at eight beginner-friendly Python projects that will help you build your skills and confidence as a programmer. These projects are designed to be fun and engaging while providing a solid foundation for more complex programming concepts.
Whether you’re interested in web development, data analysis, or simply want to learn a new skill, these projects will help you take your Python skills to the next level. So let’s dive in and unlock your Python potential with these exciting projects!
Challenge 1: Tic-Tac-Toe Game
Tic-Tac-Toe is a classic game that is simple to understand and implement, making it a great project for beginners to work on. The objective of this project is to build a Tic-Tac-Toe game in Python that can be played by two people.
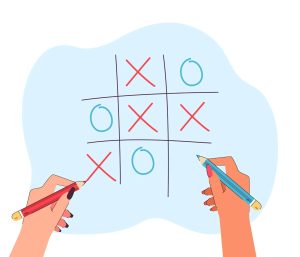
Step-by-step instructions for building the game:
- Create a basic structure for the Tic-Tac-Toe board using a nested list. Each element in the list represents a cell on the board, and can be initialized as an empty string.
- Create a function to display the Tic-Tac-Toe board on the console. This function should take the nested list as an input and display it in a visually appealing way.
- Create a function to handle player moves. This function should take the current player’s symbol, and the coordinates of their move as input, and update the Tic-Tac-Toe board accordingly.
- Create a function to check for a win. This function should check for three consecutive symbols (either “X” or “O”) in a row, column or diagonal.
- Create a function to handle the game loop. This function should handle the flow of the game, including displaying the board, getting player input, and checking for a win.
- Finally, call the game loop function to start the game.
Tips and best practices for working with Python:
- Use clear and descriptive variable names to make your code more readable.
- Use comments to explain the purpose of different sections of your code.
- Test your code frequently to catch and fix bugs early.
- Use functions to break down your code into smaller, more manageable chunks.
Here’s an example of sample code for reference:
# Tic-Tac-Toe Game in Python
def print_board(board):
"""
Print the current state of the Tic-Tac-Toe board.
"""
print("-------------")
for i in range(3):
print("|", end=" ")
for j in range(3):
print(board[i][j], "|", end=" ")
print()
print("-------------")
def get_move(player):
"""
Ask the player for their next move.
"""
print(f"Player {player}, make your move (row, col): ")
row = int(input())
col = int(input())
return (row, col)
def is_valid_move(board, move):
"""
Check if the given move is valid.
"""
row, col = move
if row < 0 or row > 2 or col < 0 or col > 2:
return False
if board[row][col] != " ":
return False
return True
def is_winner(board, player):
"""
Check if the given player has won the game.
"""
for i in range(3):
if board[i][0] == player and board[i][1] == player and board[i][2] == player:
return True
if board[0][i] == player and board[1][i] == player and board[2][i] == player:
return True
if board[0][0] == player and board[1][1] == player and board[2][2] == player:
return True
if board[0][2] == player and board[1][1] == player and board[2][0] == player:
return True
return False
def is_tie(board):
"""
Check if the game has ended in a tie.
"""
for i in range(3):
for j in range(3):
if board[i][j] == " ":
return False
return True
def play_game():
"""
Play a game of Tic-Tac-Toe.
"""
board = [[" ", " ", " "], [" ", " ", " "], [" ", " ", " "]]
players = ["X", "O"]
current_player = 0
while True:
print_board(board)
move = get_move(players[current_player])
if not is_valid_move(board, move):
print("Invalid move. Try again.")
continue
row, col = move
board[row][col] = players[current_player]
if is_winner(board, players[current_player]):
print_board(board)
print(f"Player {players[current_player]} wins!")
break
if is_tie(board):
print_board(board)
print("Tie game!")
break
current_player = (current_player + 1) % 2
play_game()
This code defines a few helper functions for printing the board, getting and validating player moves, checking for a winner or tie, and playing the game itself. The play_game function initializes the game state, then loops until there is a winner or tie, prompting each player for their move and updating the board accordingly.
Click here to check the working of the mentioned as above code.
Tic Tac Toe Game Code Using Bito
Bito’s expertise in generating and explaining Python code allows you to easily understand the code for your game. Just provide Bito with the command to generate the code, and You’ll see the code right in front of your eyes. Check out the screenshot below to see Bito’s powerful code generation in action.
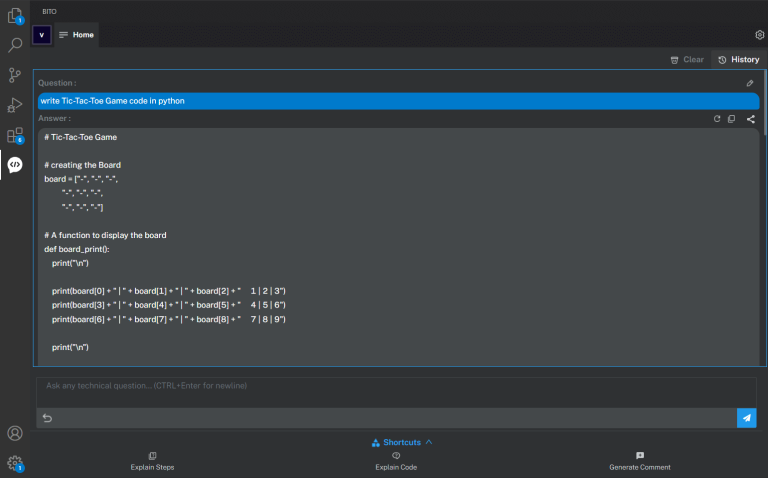
Explanation:
We selected the Tic Tae Toe game code and clicked on the ‘Explain Code’ button. Within seconds, Bito provided us with a comprehensive explanation of the code, breaking it down into easy-to-understand steps. Take a look at the image below to see Bito in action. With Bito as your coding assistant, unraveling the complexities of code has never been more effortless!
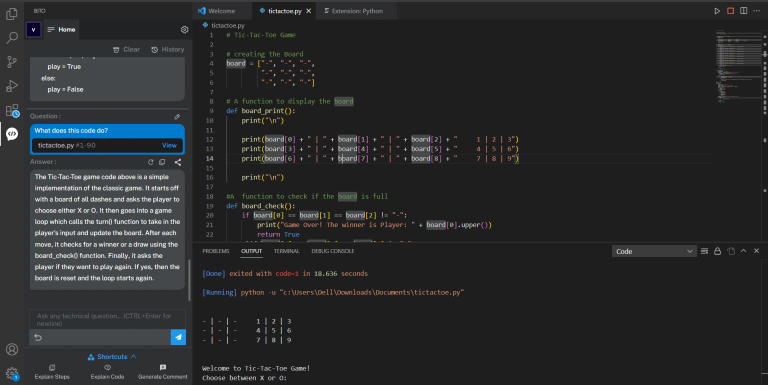
Challenge 2: Weather App
The Weather App project is a great way to learn about working with APIs in Python. The objective of this project is to build a command-line application that allows users to enter a location and get the current weather information for that location.
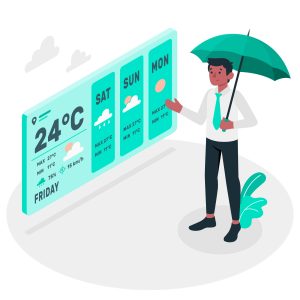
Step-by-step instructions for building the app:
- Research and find a weather API that provides current weather information in a format that is easy to work with in Python. Some popular options include OpenWeatherMap and Weather Underground.
- Sign up for an API key to access the weather data.
- Use the requests library in Python to make a GET request to the weather API, passing in the location and API key as parameters.
- Parse the JSON data returned by the API to extract the relevant information, such as temperature, humidity, and weather conditions.
- Use the print() function to display the weather information to the user in a clear and easy-to-read format.
- Use a while loop to keep the application running until the user chooses to exit.
Tips and best practices for working with Python and APIs:
- Read the API documentation carefully to understand how to make requests and what data is available.
- Use the requests library to make HTTP requests and handle the responses.
- Use the json library to parse JSON data.
- Check the API’s rate limits and make sure your code doesn’t exceed them.
- Always handle errors and unexpected responses gracefully to provide a good user experience.
Here’s an example of sample code for reference:
import requests
# API key for OpenWeatherMap
API_KEY = "your_api_key_here"
def get_weather(city_name):
"""
Get weather information for a city from OpenWeatherMap API.
"""
# Make API call to OpenWeatherMap
url = f"http://api.openweathermap.org/data/2.5/weather?q={city_name}&appid={API_KEY}&units=metric"
response = requests.get(url)
# Parse response data
data = response.json()
if data["cod"] != 200:
# Error occurred
print(f"Error: {data['message']}")
return
# Print weather information
weather_desc = data["weather"][0]["description"]
temp = data["main"]["temp"]
feels_like = data["main"]["feels_like"]
humidity = data["main"]["humidity"]
print(f"Current weather in {city_name}: {weather_desc}")
print(f"Temperature: {temp} °C")
print(f"Feels like: {feels_like} °C")
print(f"Humidity: {humidity}%")
# Example usage
get_weather("London")
This code defines a get_weather function that takes a city name as input, makes a request to the OpenWeatherMap API, and prints out the current weather information for that city. You’ll need to replace your_api_key_here with your actual OpenWeatherMap API key in order for this code to work. You can sign up for a free API key at open weather map.
Weather App Code Using Bito
Check out the picture below! We commanded Bito to generate the weather app code in Python, and it delivered flawlessly.
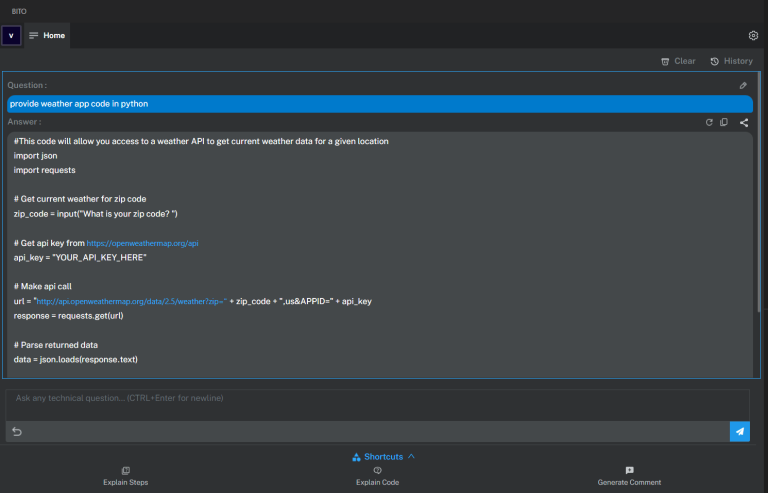
Explanation:
Check out the picture below where we selected the code and clicked on the “explain code” button to obtain a thorough explanation.
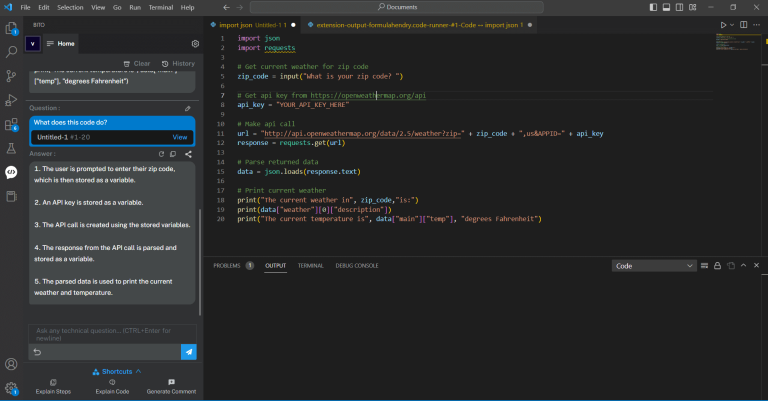
Challenge 3: To-Do List App
The To-Do List App project is a great way to learn about working with databases in Python. The objective of this project is to build a command-line application that allows users to create, read, update, and delete items from a to-do list.
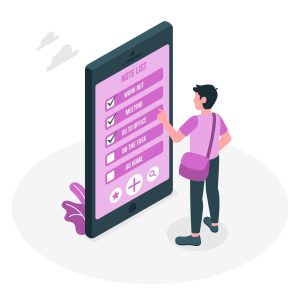
Step-by-step instructions for building the app:
- Research and decide on a database to use, such as SQLite or MySQL.
- Use the sqlite3 or mysql-connector-python library to connect to the database and create a table to store the to-do list items.
- Use the appropriate SQL commands to insert, select, update, and delete items from the table.
- Use a while loop to keep the application running until the user chooses to exit.
- Implement a menu-driven interface, allowing the user to perform CRUD operations on the to-do list.
Tips and best practices for working with Python and databases:
- Use parameterized queries to prevent SQL injection attacks.
- Always commit your transactions after making changes to the database.
- Handle database errors gracefully and provide useful error messages to the user.
- Use a library or ORM (Object-relational mapper) such as SQLAlchemy to make working with databases more pythonic and less verbose.
Here’s an example of sample code for reference:
# To-Do List App in Python
# Define a list to hold the tasks
tasks = []
# Function to add task to the list
def add_task():
task = input("Enter the task: ")
tasks.append(task)
print("Task added successfully!")
# Function to view all tasks in the list
def view_tasks():
if len(tasks) == 0:
print("No tasks found.")
else:
print("Tasks:")
for task in tasks:
print("- " + task)
# Function to remove task from the list
def remove_task():
task = input("Enter the task to be removed: ")
if task in tasks:
tasks.remove(task)
print("Task removed successfully!")
else:
print("Task not found.")
# Main function to run the app
def main():
while True:
print("\nTo-Do List App\n")
print("1. Add Task")
print("2. View Tasks")
print("3. Remove Task")
print("4. Exit")
choice = input("Enter your choice: ")
if choice == "1":
add_task()
elif choice == "2":
view_tasks()
elif choice == "3":
remove_task()
elif choice == "4":
print("Thank you for using the app!")
break
else:
print("Invalid choice. Please try again.")
# Run the app
if __name__ == "__main__":
main()
This implementation allows the user to add, view, and remove tasks from a To-Do List. The app uses a while loop to continuously prompt the user for input until they choose to exit. The tasks are stored in a list, and the add, view, and remove functions manipulate this list accordingly.
Click here to check the working of the mentioned as above code.
To-Do List App Code Using Bito
In the picture below, you can see that we gave the command to Bito to generate the code for a to-do list app in Python, and Bito successfully completed the task. It’s amazing how quickly Bito can generate code, making our development process smoother and more efficient.
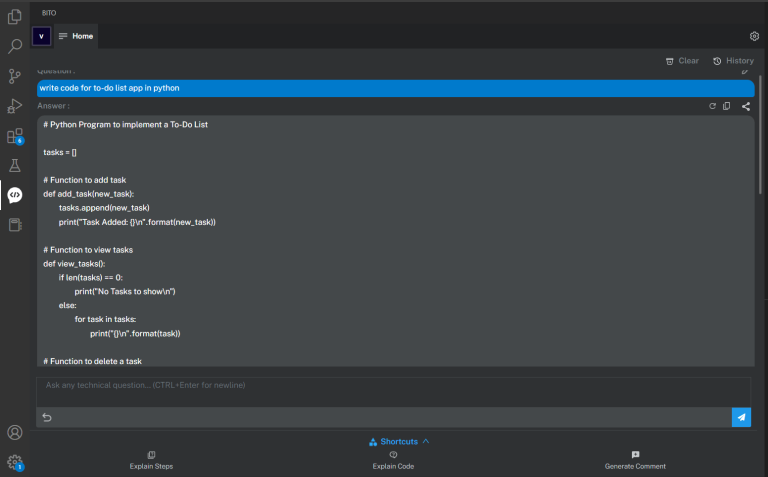
Explanation:
In the picture below, Bito is providing an informative explanation of the Weather App’s code.
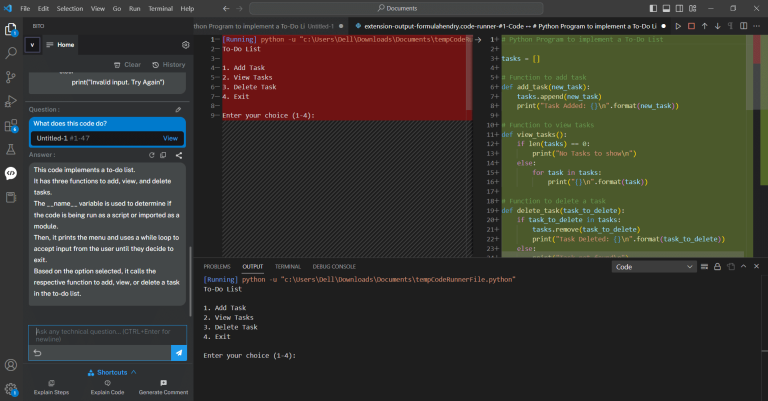
Challenge 4: Number Guessing Game
The Number Guessing Game project is a great way to learn about working with loops in Python. The objective of this project is to build a game that randomly generates a number between 1 and 100, and allows the user to guess the number. The user will be given feedback on their guesses, and will continue guessing until they correctly guess the number.
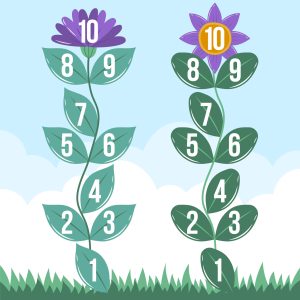
Step-by-step instructions for building the game:
- Generate a random number between 1 and 100 using the random module.
- Use a while loop to keep the game running until the user correctly guesses the number.
- Within the while loop, prompt the user to make a guess, and use an if-elif-else statement to provide feedback on their guess (e.g. “Too low”, “Too high”, “Correct!”)
- After the user correctly guesses the number, display a message indicating the number of guesses it took them.
- Optionally, allow the user to play again by using a nested loop.
Tips and best practices for working with Python and loops:
- Make sure to include a way to exit the loop, such as a flag variable or a break statement.
- Keep track of the number of iterations through the loop to provide feedback to the user (e.g. the number of guesses it took them to correctly guess the number)
- Use the range function to iterate over a set of numbers, rather than using a while loop with a counter variable.
- Nest loops when needed, but be mindful of indentation and scoping to avoid confusion.
Here’s an example of sample code for reference:
import random
# generate a random number between 1 and 100
secret_number = random.randint(1, 100)
# initialize the number of tries
num_of_tries = 0
# greet the player
print("Welcome to the number guessing game!")
print("I'm thinking of a number between 1 and 100.")
# loop until the player guesses the correct number or runs out of tries
while num_of_tries < 10:
# prompt the player to guess the number
guess = int(input("Take a guess: "))
# increment the number of tries
num_of_tries += 1
# check if the guess is correct
if guess == secret_number:
print("Congratulations! You guessed the number in", num_of_tries, "tries.")
break
elif guess < secret_number:
print("Your guess is too low.")
else:
print("Your guess is too high.")
# if the player runs out of tries without guessing the correct number, show the secret number
if num_of_tries == 10:
print("Sorry, you didn't guess the number in time. The secret number was", secret_number, ".")
In this game, the player has 10 tries to guess a randomly generated number between 1 and 100. After each guess, the game tells the player if the guess is too high or too low. If the player guesses the correct number, the game congratulates them and shows the number of tries it took to guess the number. If the player runs out of tries without guessing the correct number, the game shows the secret number.
Click here to check the working of the mentioned as above code.
Number Guessing Game Code Using Bito
Check out the picture below where we commanded Bito to generate Python code for a Number Guessing Game, and Bio did it easily.
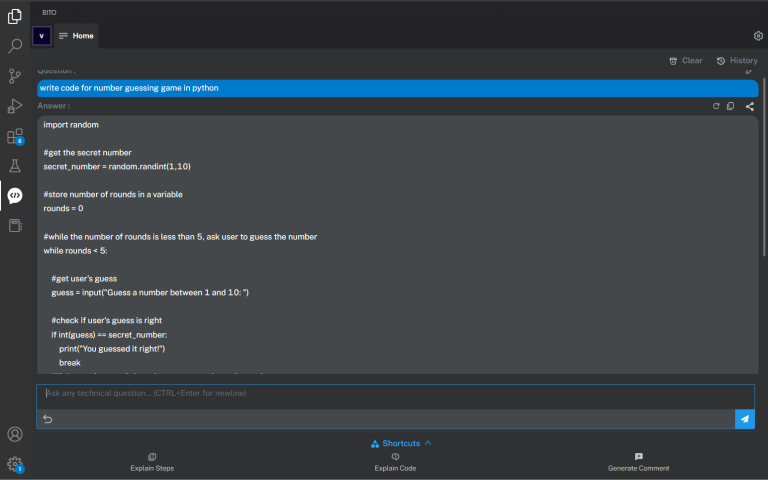
Explanation:
Check out the picture below where Bito explains the Number Guessing Game code in an easy-to-understand way.
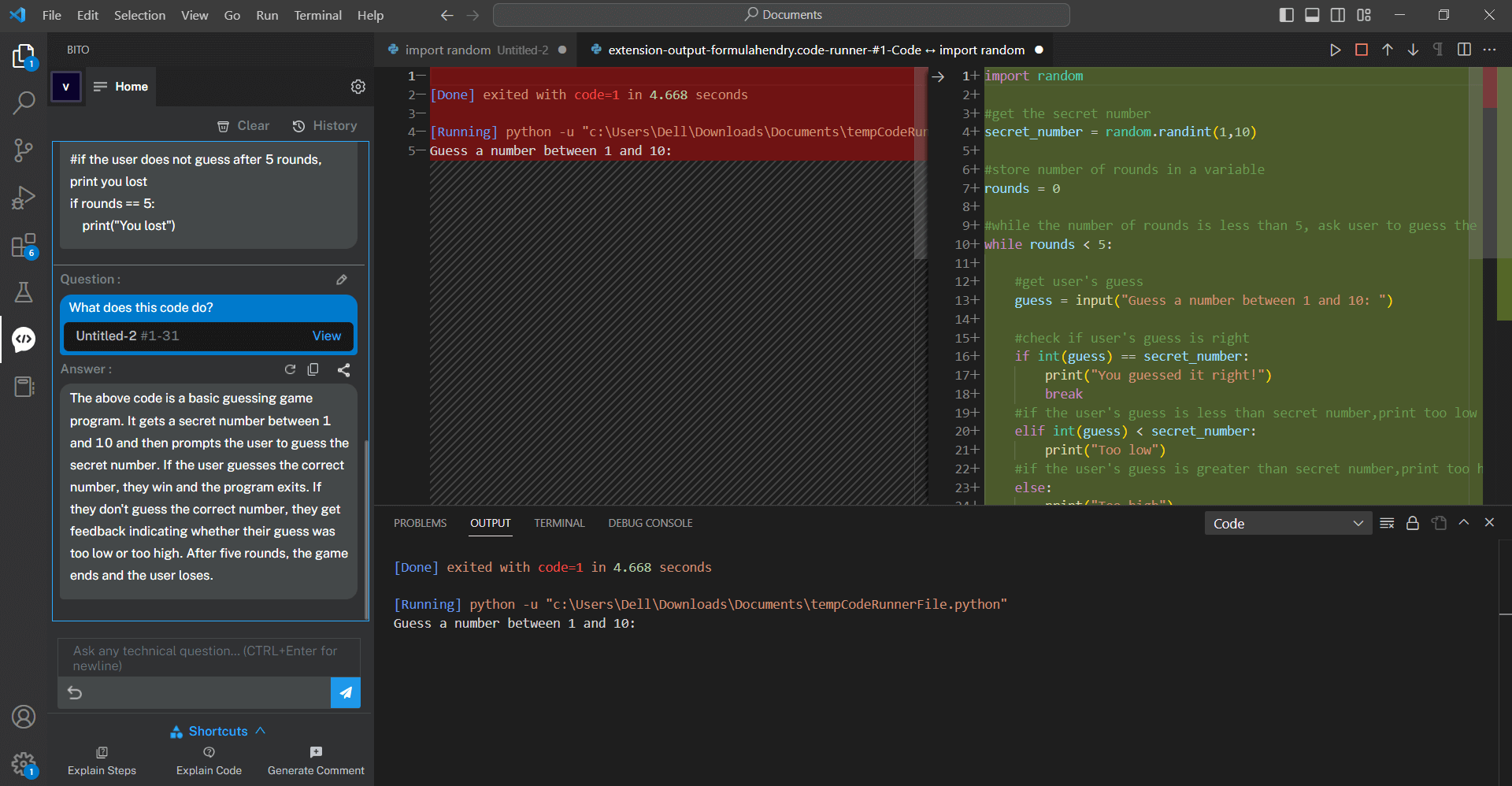
Challenge 5: Mad Libs Game
The Mad Libs Game project is a fun way to learn about working with strings in Python. The objective of this project is to build a game that prompts the user for various words or phrases, and then inserts those words into a pre-written story to create a silly, new version of the story.
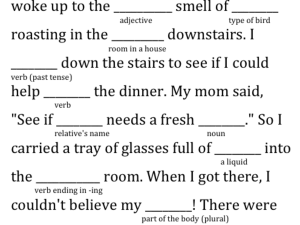
Step-by-step instructions for building the game:
- Create a string that contains a simple story with several blank spaces (e.g. “Once upon a time, there was a ___ who lived in a ___.”)
- Use string formatting to replace the blank spaces with words or phrases that the user inputs.
- Use a loop to prompt the user for each word or phrase needed to complete the story.
- After all the words have been entered, print the completed story.
- Optionally, allow the user to play again by using a nested loop.
Tips and best practices for working with Python and strings:
- Use string formatting to insert user input into a string, rather than concatenating strings together.
- Use the .format() method to insert variables into strings, rather than using string concatenation.
- Use the .strip() method to remove leading and trailing whitespace from user input.
- When storing user input, use a variable name that is descriptive and meaningful.
Here’s an example of sample code for reference:
print("Welcome to Mad Libs!")
# Get inputs from user
noun1 = input("Enter a noun: ")
verb1 = input("Enter a verb: ")
adjective1 = input("Enter an adjective: ")
noun2 = input("Enter another noun: ")
verb2 = input("Enter another verb: ")
adjective2 = input("Enter another adjective: ")
# Print out the story with the user's inputs
print("Once upon a time, there was a " + adjective1 + " " + noun1 + " who loved to " + verb1 + ".")
print("One day, while " + verb2 + "ing " + noun2 + ", the " + noun1 + " met a " + adjective2 + " stranger.")
print("The stranger invited the " + noun1 + " to join a secret club for people who love to " + verb2 + ".")
print("Excitedly, the " + noun1 + " accepted the invitation and became a member of the club.")
print("The End.")
This code prompts the user for various inputs (a noun, verb, and adjective), and then uses those inputs to fill in the blanks in a pre-written story. Finally, it prints out the completed story with the user’s inputs. You can customize the story and prompts to your liking!
Click here to check the working of the above mentioned code.
Mad Libs Game Code Using Bito
Take a look at the picture below where Bito effortlessly generated Python code for a Mad Libs Game upon our request.
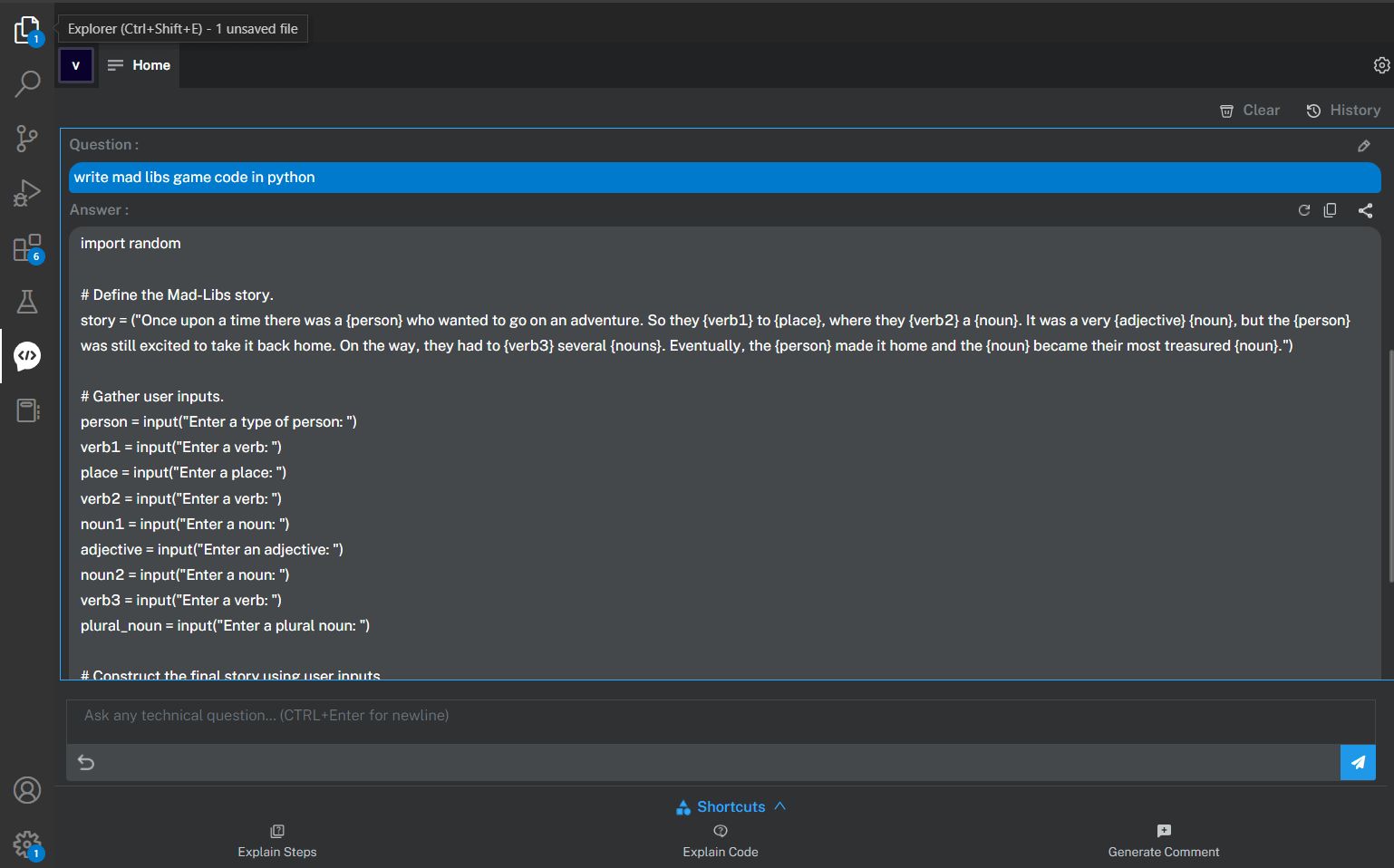
Explanation:
Check out the picture below where Bito explains the Mad Libs Game code in a simple and easy-to-follow way. With Bito’s help, you’ll have no trouble creating your own game!
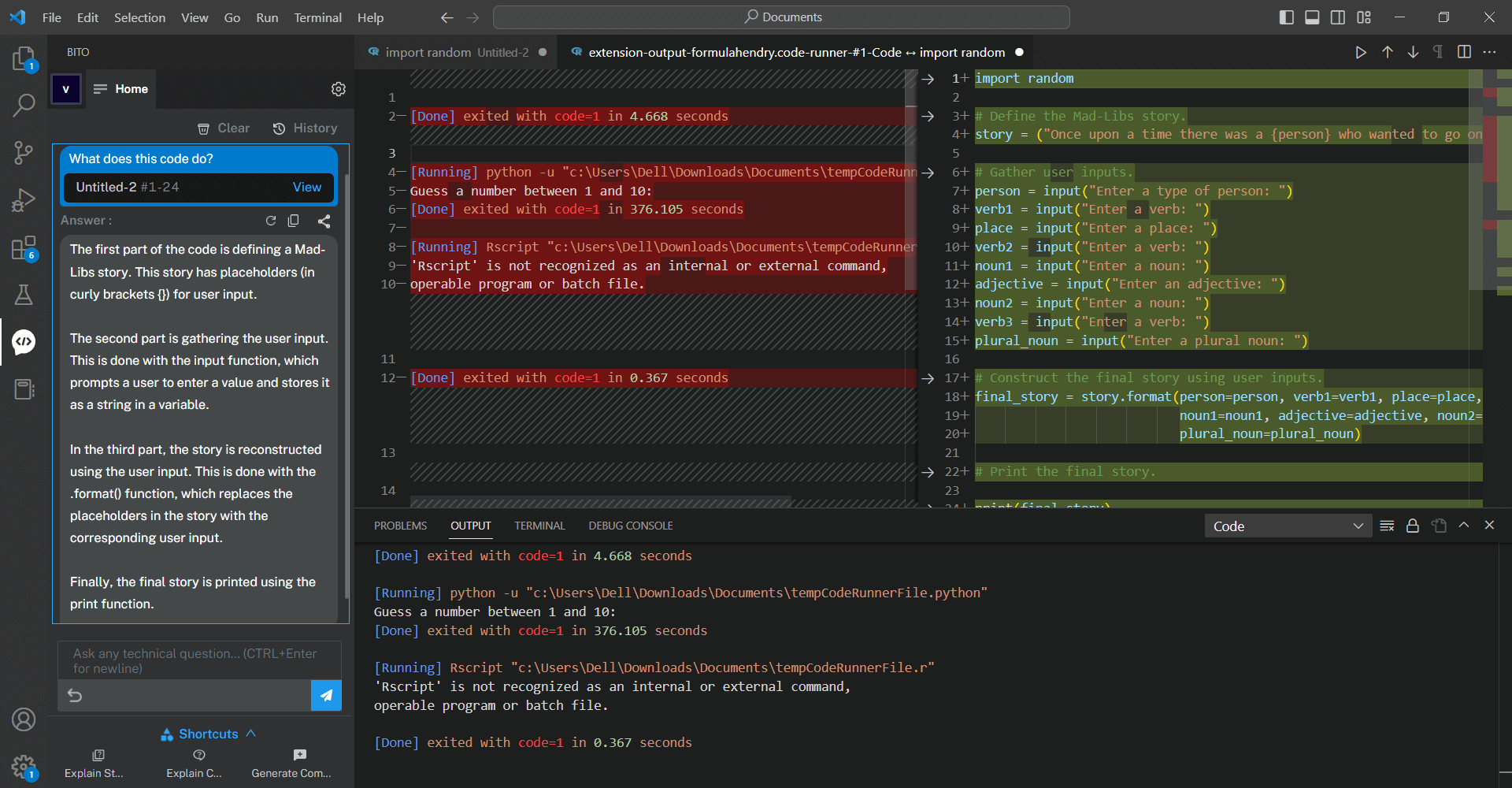
Challenge 6: Rock, Paper, Scissors Game
The Rock, Paper, Scissors Game project is a great way to learn about basic control flow in Python. The objective of this project is to build a simple game where the user plays against the computer and the winner is determined based on the rules of the game.
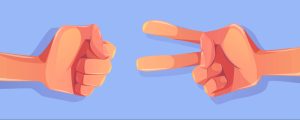
Step-by-step instructions for building the game:
- Create a list of the three possible choices (rock, paper, scissors).
- Use the random module to generate a random choice for the computer.
- Prompt the user for their choice.
- Use if-elif-else statements to determine the winner based on the rules of the game.
- Print the results.
- Optionally, you can use a loop to keep playing until the user wants to quit.
Tips and best practices for working with Python and basic control flow:
- Use if-elif-else statements to make decisions based on the input
- Use the random module to generate random numbers or choices.
- Use while loops to repeatedly execute a block of code.
- Use the input() function to get user input and the print() function to output results
- Print the results.
Here’s an example of sample code for reference:
import random
# Define the options
options = ["rock", "paper", "scissors"]
# Define the function to determine the winner
def determine_winner(player_choice, computer_choice):
if player_choice == computer_choice:
return "Tie"
elif player_choice == "rock" and computer_choice == "scissors":
return "Player wins"
elif player_choice == "paper" and computer_choice == "rock":
return "Player wins"
elif player_choice == "scissors" and computer_choice == "paper":
return "Player wins"
else:
return "Computer wins"
# Play the game
while True:
# Get the player's choice
player_choice = input("Choose rock, paper, or scissors: ").lower()
while player_choice not in options:
player_choice = input("Invalid choice. Choose rock, paper, or scissors: ").lower()
# Get the computer's choice
computer_choice = random.choice(options)
# Determine the winner
winner = determine_winner(player_choice, computer_choice)
# Print the results
print(f"Player chose {player_choice}, computer chose {computer_choice}. {winner}!\n")
# Ask if the player wants to play again
play_again = input("Play again? (y/n): ").lower()
while play_again != "y" and play_again != "n":
play_again = input("Invalid choice. Play again? (y/n): ").lower()
if play_again == "n":
break
When you run the code, it will ask the player to choose rock, paper, or scissors. The computer will then randomly choose rock, paper, or scissors. The function determine_winner will determine who won the game, and the results will be printed to the console. The player will then be asked if they want to play again. If they do, the game will start over. If they don’t, the program will exit.
Note that this code is a basic example and can be improved in many ways. For example, you could add error handling for when the player inputs something other than “y” or “n”, or you could add a way to keep track of the score.
Click here to check the working of the mentioned as above code.
Rock, Paper, Scissors Game Code Using Bito
See the picture below where Bito creates a Rock, Paper, Scissors Game code for us with ease. It’s impressive how skilled Bito is!
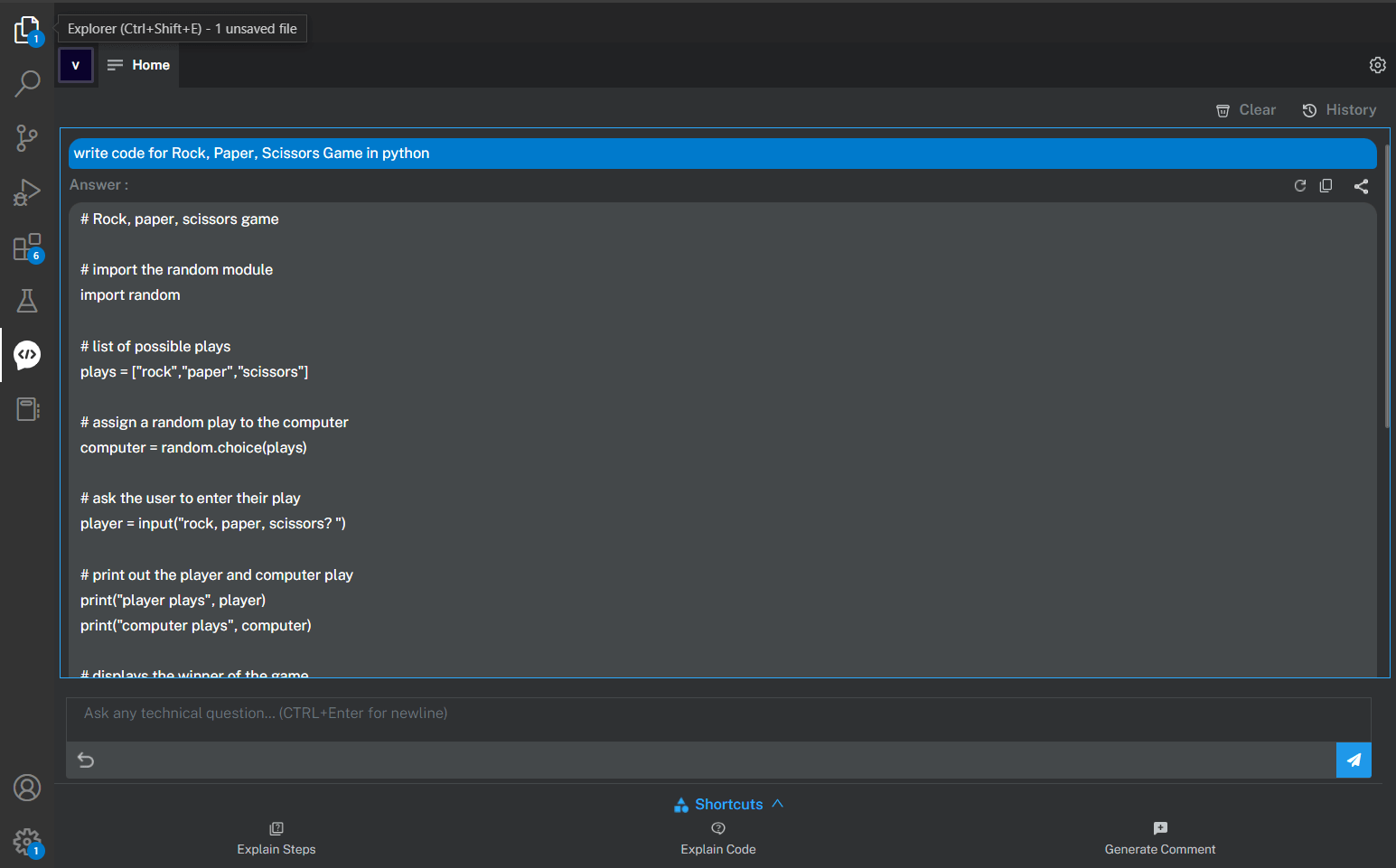
Explanation:
Take a look at the picture below where Bito explains the Rock, Paper, Scissors Game code in a simple way.
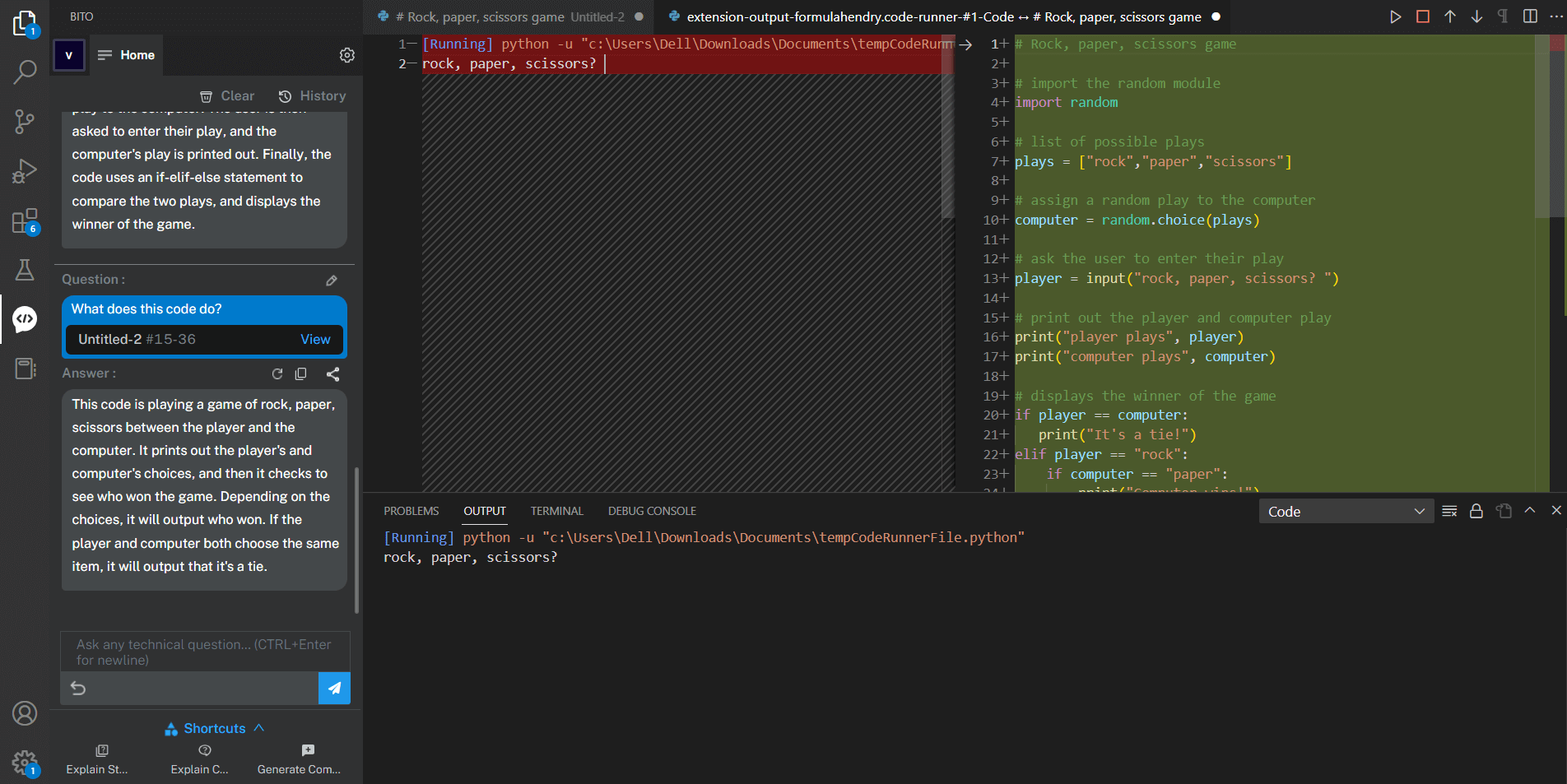
Challenge 7: Hangman Game
The Hangman game project provides an opportunity to practice problem-solving skills and logical thinking, as the player has to guess the word based on the clues given by the game. It also allows for creativity and customization, as one can modify the game rules, add new features, or design a different user interface .
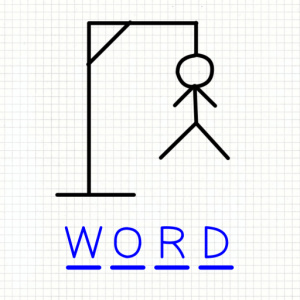
Step-by-step instructions for building the game:
- Choose a word to use as the secret word.
- Create a list of underscores the same length as the secret word to represent the unguessed letters.
- Use a loop to repeatedly ask the user for a letter and check if it is in the secret word.
- If the letter is in the secret word, update the list of underscores with the correctly guessed letters.
- If the letter is not in the secret word, decrease the number of remaining attempts.
- End the game when the user has correctly guessed all the letters in the secret word or when the number of remaining attempts is zero.
Tips and best practices for working with Python and basic string manipulation:
- Use the in keyword to check if a letter is in a word
- Use the .join() method to join a list of strings into a single string
- Use the .replace() method to replace characters in a string
- Use the .format() method to insert variables into a string
Here’s an example of sample code for reference:
import random
def hangman():
word_list = ["python", "java", "kotlin", "javascript"]
chosen_word = random.choice(word_list)
word_length = len(chosen_word)
attempts = 6
guessed_letters = []
hidden_word = list("-" * word_length)
print("H A N G M A N\n")
while attempts > 0:
print("".join(hidden_word))
guess = input("Input a letter: ")
if guess in guessed_letters:
print("You already typed this letter")
elif len(guess) != 1:
print("You should input a single letter")
elif not guess.isalpha() or guess.isupper():
print("It is not an ASCII lowercase letter")
elif guess in chosen_word:
for i in range(word_length):
if chosen_word[i] == guess:
hidden_word[i] = guess
guessed_letters.append(guess)
else:
print("No such letter in the word")
guessed_letters.append(guess)
attempts -= 1
if "-" not in hidden_word:
print("\nYou guessed the word!")
print("You survived!")
return
print()
print("You are hanged!")
return
hangman()
This implementation chooses a word at random from a pre-defined list, sets a maximum number of attempts to 6, and allows the user to input a single lowercase letter at a time. The game checks for valid input, whether the guess is in the word, and updates the hidden word accordingly. If the player wins, the game congratulates them; if they lose, the game ends and displays a message indicating they were hanged
Click here to check the working of the above-mentioned code.
Hangman Game Code Using Bito
Take a look at the picture below where Bito expertly generates a Hangman Game code on our request.
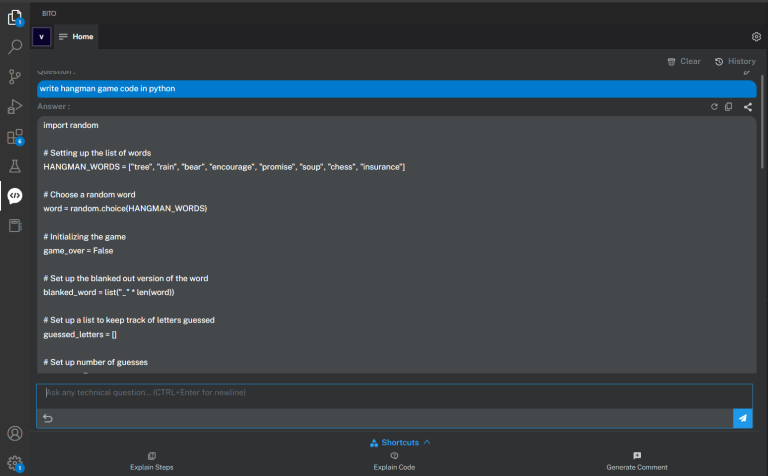
Explanation:
Look at the picture below where we clicked ‘Explain Code’ and Bito simplified the Hangman Game code.
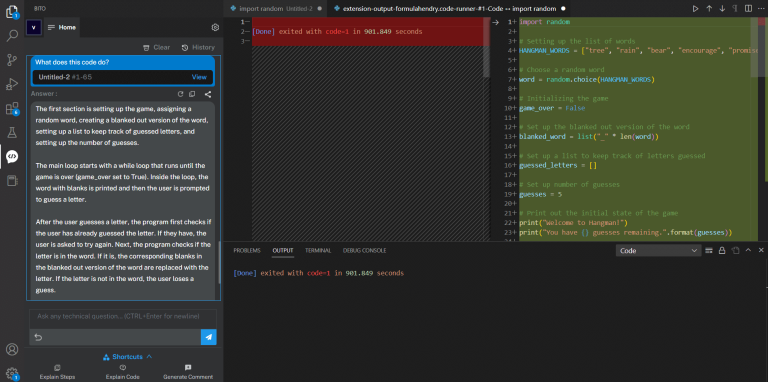
Challenge 8: Simple Calculator
By building a simple calculator project in Python, you can learn programming basics such as variables, data types, input/output, conditional statements, functions, error handling, testing, and debugging. This project will help you gain hands-on experience with programming concepts and develop your coding skills. You will learn how to use variables, prompt for user input, display output, make decisions based on user input, define and use functions to perform calculations, handle errors, test your code, and debug any errors that arise.
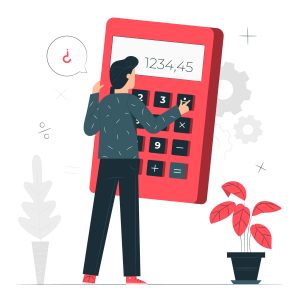
Step-by-step instructions for building the calculator:
- Define four functions for addition, subtraction, multiplication, and division.
- Print a message to the user asking them to select an operation.
- Print a list of the available operations for the user to choose from.
- Prompt the user to enter their choice of operation.
- Prompt the user to enter two numbers.
- Use conditional statements to check the user’s choice of operation and call the appropriate function.
- Display the result of the calculation to the user.
Tips and best practices for working with Python and basic mathematical operations:
- Use the input() function to ask the user for input.
- Use the int() or float() functions to convert user input to a numerical data type.
- Use the +, -, *, and / operators for addition, subtraction, multiplication, and division respectively.
- Use the % operator for modulus operation
- Use the ** operator for exponentiation
Here’s an example of sample code for reference:
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
return x / y
print("Select operation.")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice (1/2/3/4): ")
num1 = int(input("Enter first number: "))
num2 = int(input("Enter second number: "))
if choice == '1':
print(num1, "+", num2, "=", add(num1, num2))
elif choice == '2':
print(num1, "-", num2, "=", subtract(num1, num2))
elif choice == '3':
print(num1, "*", num2, "=", multiply(num1, num2))
elif choice == '4':
print(num1, "/", num2, "=", divide(num1, num2))
else:
print("Invalid input")
In this code, we define four functions for addition, subtraction, multiplication, and division. We then prompt the user to select an operation and enter two numbers. Based on the user’s choice of operation, we call the appropriate function and display the result to the user. If the user inputs an invalid choice, we display an error message.
Click here to check the working of the above mentioned code.
Simple Calculator Code Using Bito
See the picture below where Bito creates a Simple Calculator code with ease upon our request.
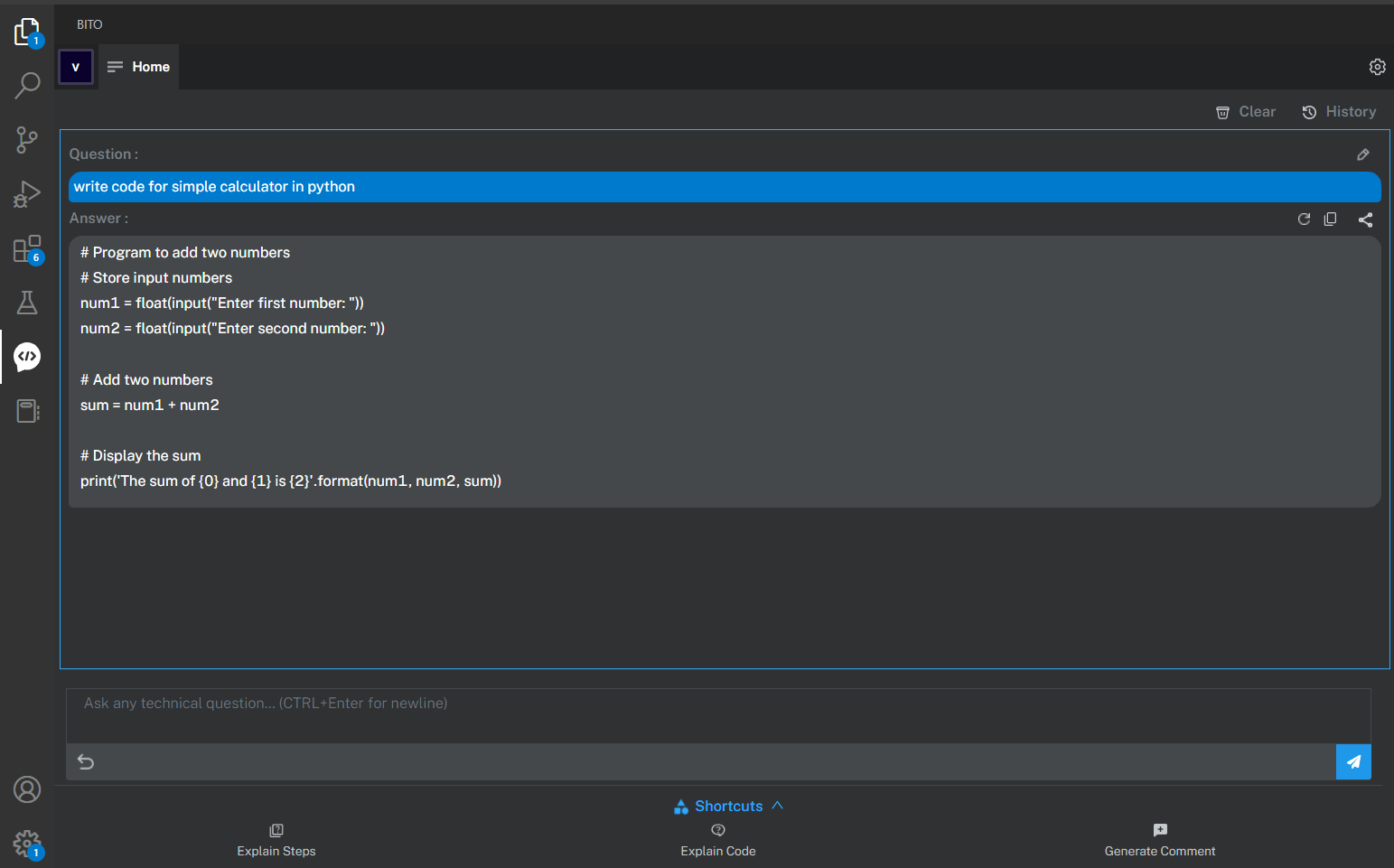
Explanation:
Look at the picture below where we clicked ‘Explain Steps’ and Bito explained how the Simple Calculator code works in a simple way.
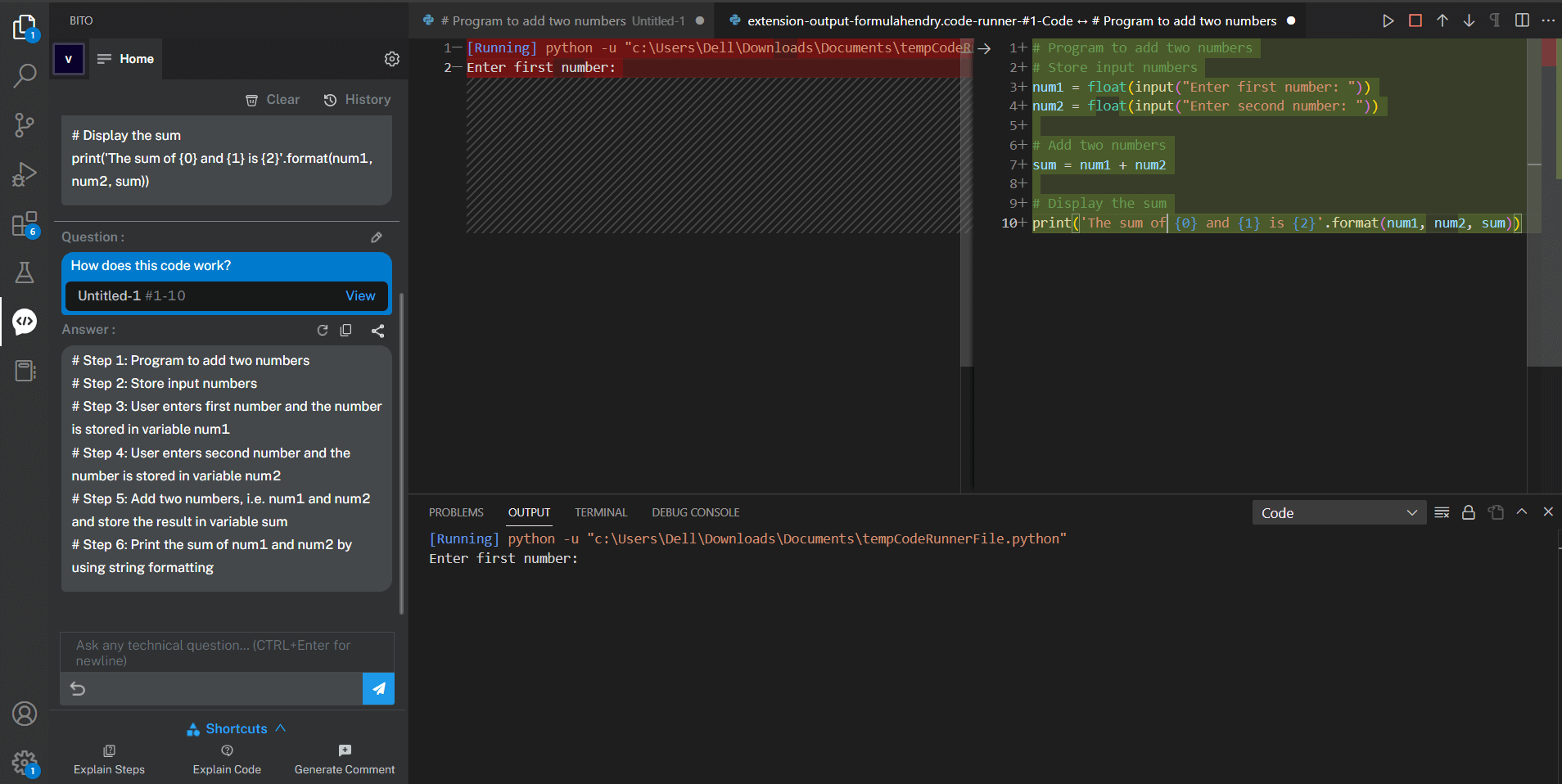
Conclusion
Congratulations on taking your first step towards mastering Python! These beginner-level projects are just the beginning of your journey, and they will give you a solid foundation to build upon.
However, why stop at just the basics? With BITO AI, you can take your Python projects to the next level and implement advanced features and functionality with ease. BITO AI is an AI-powered Python code assistant that can help you write faster, smarter, and better code. From generating code snippets to suggesting alternative solutions, BITO AI makes coding easier and more efficient.
Imagine being able to focus on the creative aspects of your project, rather than getting bogged down in the technical details. With BITO AI, you can do just that. By automating repetitive tasks and streamlining your coding workflow, BITO AI allows you to create even more amazing games and applications.
Don’t wait any longer to unlock the full potential of your coding skills. Try BITO AI today and see how it can revolutionize the way you code. Head over to https://bito.ai/ and install it now to experience the power of AI-assisted programming for yourself.